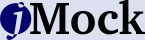
Firstly, I would like to provide a small fix to the code provided in the previous entry:
JMock for TestNG (or JUnit-free JMock)
/**
* Verify the expected behavior for the mocks registered by the current thread and
* also releases them.
*/
public static synchronized void verifyAndRelease() {
Thread currentThread= Thread.currentThread();
List mocks = s_mockObjects.get(currentThread);
if(null != mocks) {
try {
for(Verifiable verifiable : mocks) {
verifiable.verify();
}
finally {
// make sure the mocks are released
mocks.clear();
s_mockObjects.put(currentThread, null);
}
}
}
The fix assures that even if the verification of a mock fails, the current mocks are released and will not remain around till the next test.
I have started to completely free
JMock of its JUnit "addiction". I have found three types of dependencies:
- classes in the hierarchy of org.junit.TestCase (3). These can be completely replaced by the class I have already posted
- usage of
org.junit.AssertionFailureException
. Having in mind that TestNG works for JVM 1.4+, we have directly used the java.lang.AssertError
, and I am thinking to do the same here
- a couple of classes extending
org.junit.Assert
(even if they aren't using much of it). Considering that TestNG propose a more readable form for assertions through org.testng.Assert, this change is quite trivial
I can confess that these changes are already available on my machine and I am already using them. Me and Joe have been discussing about the possibility to integrate these changes directly in JMock. If this will not happen, than I will most probably include the code in
TestNG.
A last point (one that I just get me burnt tonite), is that
JMock doesn't check (or I haven't figured how to make it) the order of expectations, so from its perspective the following code is same correct (or wrong):
[...]
mock.expect(once()).method("methodOne");
mock.expect(once()).methog("methodTwo");
[...]
mock.verify();
and
[...]
mock.expect(once()).method("methodTwo");
mock.expect(once()).methog("methodOne");
[...]
mock.verify();
will both pass or fail disregarding the real order of invocation. I agree that in most of the cases, JMock behavior is enough good, but not having a way to force the order of invocation is in my opinion a missing feature. I am gonna try to add this feature to my code.
2 comments:
hi alex,
i think there is a way to force the order of invocation, please take a look at IdentityBuilder.id() and MatchBuilder.after() method.
teh following code force methodTwo to be invoked after methodOne:
mock.expects(once()).method("methodOne").id("one");
mock.expects(once()).methog("methodTwo").after("one");
/iulian
Thanks for the pointer. I will check that. However, at first glance this form is loosing a little bit from the readability of JMock API.
./alex
--
.w( the_mindstorm )p.
Post a Comment